자바로 xml 공공데이터를 가져와서 파싱 해봅시다.
공공데이터
공공데이터는 근로복지공단 질병판정서 조회 서비스를 사용할 겁니다.
활용신청을 하면 활용신청 상세기능정보에서 심의결과 유형조회가 있습니다. 이 데이터를 가져오겠습니다
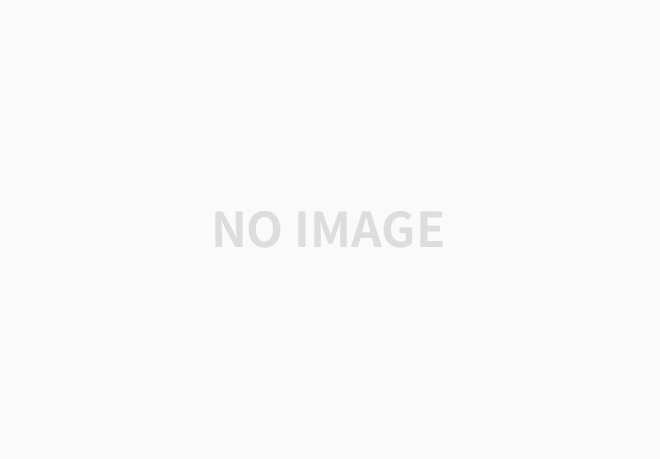
심의결과 유형조회에서 자신의 인증키를 넣고 미리보기를 누르면 xml 코드가 있는 웹사이트가 뜹니다.
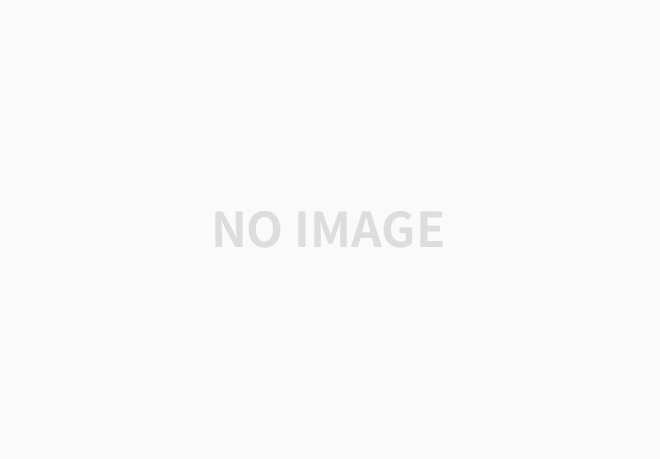
xml 코드를 보면 맨 위에 response가 있고 그다음 header랑 body태그가 있습니다.
그리고 header태그 밑에 resultCode랑 resultMsg태그가 있습니다. 또한 body태그도 items태그 그 안에 item 그리고 numOfRows태그랑 pageNo태그 totalCount태그가 있습니다.
이 xml코드를 자바 모델클래스로 변환할겁니다.
https://json2csharp.com/code-converters/xml-to-java
아래 사이트를 가면 xml 코드를 입력하는 부분이 나옵니다.
xml 코드 자바 모델클래스로 변환
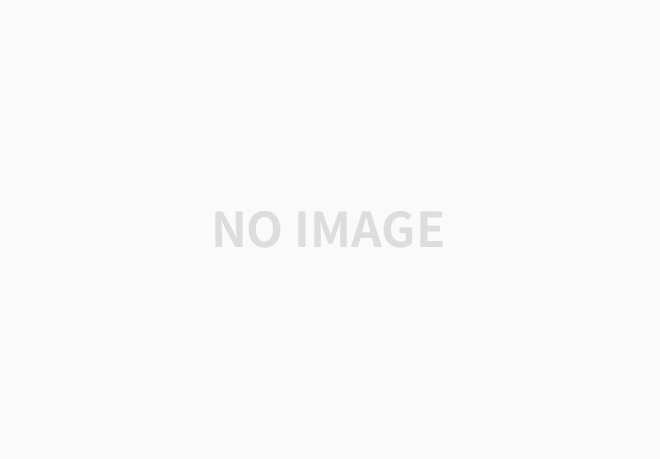
Use properties를 체크하면 get set코드가 나옵니다. xml코드를 붙여넣기한 후 Convert를 누르면 자바 모델클래스가 생성됩니다.
Zip as Files를 누르면 자바 클래스 압축 파일이 나오는데 압축을 풀어주고 파일을 다 복사해서 안드로이드 스튜디오 프로젝트 파일에 붙여넣기 해줍시다.
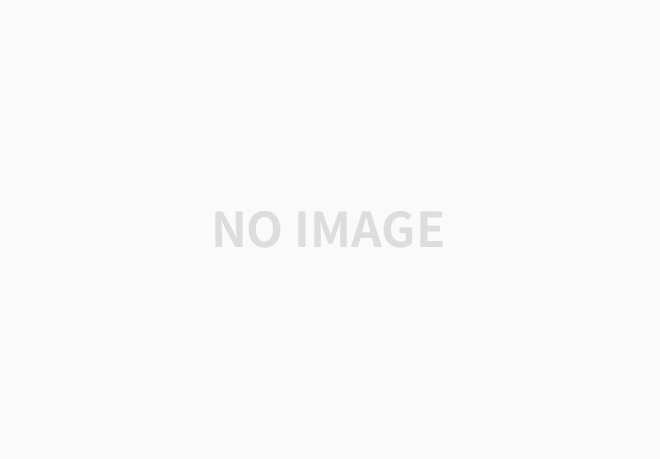
위 사이트로 xml코드를 자바 모델클래스로 변환하였습니다. 이제 안드로이드 스튜디오로 돌아가서 코드를 작성해줍시다.
implementation
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.tickaroo.tikxml:annotation:0.8.13'
implementation 'com.tickaroo.tikxml:core:0.8.13'
implementation 'com.tickaroo.tikxml:retrofit-converter:0.8.13'
annotationProcessor 'com.tickaroo.tikxml:processor:0.8.13'
위 부분을 implementation 해줍시다.
MainActivity.java
package com.grandera.tistory;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.widget.TextView;
import com.tickaroo.tikxml.TikXml;
import com.tickaroo.tikxml.retrofit.TikXmlConverterFactory;
import java.io.UnsupportedEncodingException;
import java.util.HashMap;
import java.util.Map;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
import retrofit2.Retrofit;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://apis.data.go.kr/B490001/jilbyeongPstateInfoService/")
.addConverterFactory(TikXmlConverterFactory.create(new TikXml.Builder().exceptionOnUnreadXml(false).build()))
.build();
ApiService service = retrofit.create(ApiService.class);
Call<response> call = service.getXmlData();
call.enqueue(new Callback<response>() {
@Override
public void onResponse(@NonNull Call<response> call, Response<response> response) {
response xmlData = response.body();
// 파싱된 XML 데이터를 처리합니다.
assert xmlData != null;
Log.v("태그","값:"+xmlData.getbody().getitems().getitem().get(0).getkinda());
}
@Override
public void onFailure(Call<response> call, Throwable t) {
// 오류 처리를 합니다.값
Log.v("태그","값:"+t.getMessage());
}
});
}
}
xml코드를 파싱해서 원하는 값인 kinda부분을 가져오겠습니다. 그리고 그 값을 Log로 띄어보겠습니다.
ApiService.java
package com.grandera.tistory;
import retrofit2.Call;
import retrofit2.http.GET;
public interface ApiService {
@GET("getSimuiResultYuhyeongPstate?serviceKey=본인의 인증키&pageNo=1&numOfRows=10")
Call<response> getXmlData();
}
꼭 본인의 인증키를 입력해 주세요
모델 클래스 어노테이션
모델 클래스에 어노테이션 부분을 각 속성에 쏵다 적어주셔야 됩니다.
여기서 @Xml, @Element, @PropertyElement를 입력할 것입니다.
@Element -> 자식이 있을경우
@Element -> 자식이 없을경우
자식이 있을경우란 body태그처럼 안에 태그가 있는 경우를 말하고
자식이 없는경우는 totalCount처럼 싱글인 경우를 말합니다.
response.java
package com.grandera.tistory;
import com.tickaroo.tikxml.annotation.Attribute;
import com.tickaroo.tikxml.annotation.Element;
import com.tickaroo.tikxml.annotation.Xml;
import retrofit2.http.Header;
@Xml(name = "response")
public class response {
public header getheader() {
return this.header; }
public void setheader(header header) {
this.header = header; }
@Element(name = "header")
header header;
public body getbody() {
return this.body; }
public void setbody(body body) {
this.body = body; }
@Element(name = "body")
body body;
}
여기서 name은 xml태그를 입력하면 됩니다. header태그라 header를 입력했습니다.
body.java
package com.grandera.tistory;
import com.tickaroo.tikxml.annotation.Element;
import com.tickaroo.tikxml.annotation.PropertyElement;
import com.tickaroo.tikxml.annotation.Xml;
@Xml(name = "body")
public class body {
public items getitems() {
return this.items; }
public void setitems(items items) {
this.items = items; }
@Element(name = "items")
items items;
public int getnumOfRows() {
return this.numOfRows; }
public void setnumOfRows(int numOfRows) {
this.numOfRows = numOfRows; }
@PropertyElement(name = "numOfRows")
int numOfRows;
public int getpageNo() {
return this.pageNo; }
public void setpageNo(int pageNo) {
this.pageNo = pageNo; }
@PropertyElement(name = "pageNo")
int pageNo;
public int gettotalCount() {
return this.totalCount; }
public void settotalCount(int totalCount) {
this.totalCount = totalCount; }
@PropertyElement(name = "totalCount")
int totalCount;
}
header.java
package com.grandera.tistory;
import com.tickaroo.tikxml.annotation.PropertyElement;
import com.tickaroo.tikxml.annotation.Xml;
@Xml(name="header")
public class header {
public int getresultCode() {
return this.resultCode; }
public void setresultCode(int resultCode) {
this.resultCode = resultCode; }
@PropertyElement(name = "resultCode")
int resultCode;
public String getresultMsg() {
return this.resultMsg; }
public void setresultMsg(String resultMsg) {
this.resultMsg = resultMsg; }
@PropertyElement(name = "resultMsg")
String resultMsg;
}
item.java
package com.grandera.tistory;
import com.tickaroo.tikxml.annotation.Element;
import com.tickaroo.tikxml.annotation.PropertyElement;
import com.tickaroo.tikxml.annotation.Xml;
@Xml(name = "item")
public class item {
public String getkinda() {
return this.kinda; }
public void setkinda(String kinda) {
this.kinda = kinda; }
@PropertyElement(name = "kinda")
String kinda;
}
items.java
package com.grandera.tistory;
import com.tickaroo.tikxml.annotation.Element;
import com.tickaroo.tikxml.annotation.Xml;
import java.util.List;
@Xml(name = "items")
public class items {
public List<item> getitem() {
return this.item; }
public void setitem(List<item> item) {
this.item = item; }
@Element(name = "item")
List<item> item;
}
실행을 해보면

잘 뜨는걸 확인할 수 있습니다.
'Coding > Android' 카테고리의 다른 글
JetPack compose 뒤로가기 버튼 이벤트 추가하기 (0) | 2023.02.23 |
---|---|
안드로이드 자바 retrofit2 json 공공데이터 가져오기 (0) | 2023.02.21 |
JetPack Compose 인텐트 액티비티 전환하기 (0) | 2023.02.18 |
JetPack Compose 구성 요소 (0) | 2023.02.18 |
jetpack compose 개요 및 구성 (0) | 2023.02.18 |